Need to interview a React developer? Check out the top 36 React interview questions and answers asked from basic to advanced levels.
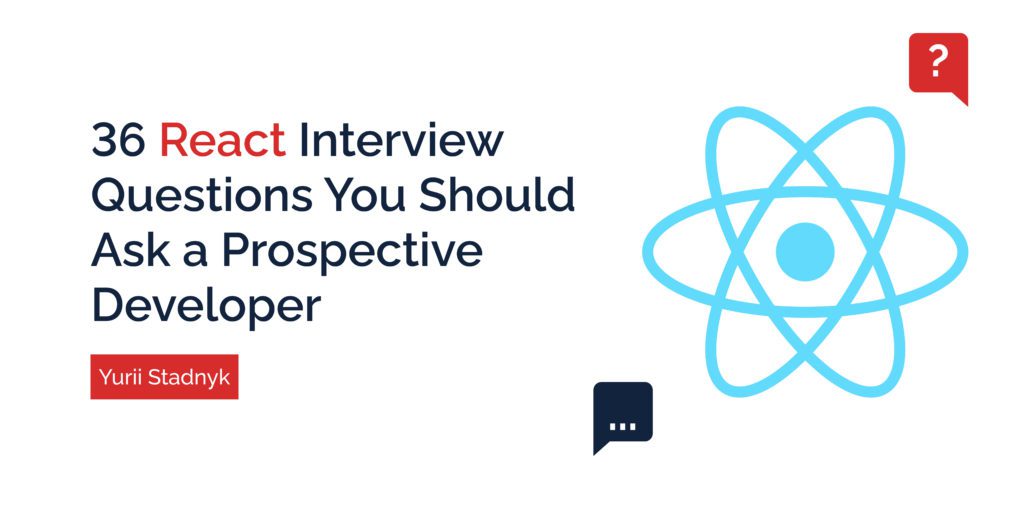
Hiring a React developer may be a trouble for someone who doesn’t know much about React. You or your non-technical HR managers are sitting there, listening to all this mumble about rendering, bundling, and routing, and trying to understand if the developer is smart enough to join the team by the look on their face.
Hopefully, you or somebody else on your team knows what React is about and can tell an amateur from a professional and knowledgeable developer. In any case, we would gladly share the thirty React interview questions that we ask when talking to candidates for this position at Keenethics. If you are well-familiar with React already, you may borrow some interesting ideas for React.js interview questions here. If you don’t know much about React, I will be happy to share everything you need for creating relevant interview questions for React. js developers.
There are two important things to keep in mind when preparing interview questions on React.js:
1. You should not limit yourself with React js interview questions and answers. Do not forget to ask about the fundamentals of programming, JavaScript, front-end development, network data transfer, security, and code testing.
2. Your questions should be ordered from the simplest to the most difficult ones.
Now, I will show you the 36 React interview questions that I recommend asking. They are split into seven blocks:
- Programming
- JavaScript
- Front-End development
- React
- Network data transfer
- Security
- Code testing
Under each block, I will tell why it is essential for the developer to know this topic. Under each question, I will explain what answer you should expect.
React Basics — React Interview Questions
Why to ask these React interview questions:
This part is the most obvious. When you interview a React developer, React is what you ask them about. Here are the top React interview questions and answers
1. What Is React? What Types of React Components Are You Familiar With?
What answer to expect:
React is “a free and open-source front-end JavaScript library for building user interfaces based on UI components” (as Wiki points out). React can be used as a base in developing SPAs or mobile apps. Besides, React is maintained by Meta (previously known as Facebook).
React has four types of components: functional components, pure components, class components, and higher-order components. Functional components take in props and return JSX. Pure components are the simplest and fastest — they don’t depend on the state of variables from the outer scope. Class components consist of a class and can use all the major React functions. Higher-order components are advanced components, and they return one or more components depending on the array data.
2. What Is The Difference Between Real DOM and Virtual DOM?
What answer to expect:
DOM stands for Document Object Model, and it is a mechanism for programs to access and update the structure, style, and contents of a document. Real DOM, also called browser DOM or HTML DOM, treats a document as a tree with its elements being nodes. Yet, real DOM is very inefficient and hard to manage. Virtual DOM is an abstract form of real DOM, a more lightweight one. All ReactJS reviews mention the fact that React uses virtual DOM as its ultimate advantage.
3. What types of hooks are there in React? How to handle the lifecycle inside the hooks properly?
What answer to expect:
Hooks are the functional components, such as useEffect, useState, useRef, useCallback. To handle a lifecycle, you use the useEffect hook.
4. How can you create high-order components in React?
What answer to expect:
A higher-order component is a function that takes one component and returns another component, a new one. As for how they are created, the candidate is expected to provide an example of the most basic technical implementation. To get a general impression of what the basic implementation of high-order components looks like, check the official React documentation.
5. What is the concept of keys in React, and why cannot we use indexes of the map as keys for dynamic lists?
What answer to expect:
Keys are the properties given to every element in an array to give them a unique ID and identify which items have changed (added/removed/re-ordered). Keys have to be static and unique. Meanwhile, a map is an iterative function. If we used map indexes as keys for dynamic lists, then the entire list would be rerendered once a change in a single element occurred.
6. How events are handled in React?
What answer to expect:
There are many different types of events: keyboard, mouse, touch, clipboard, form events, and a lot more. The process of handling events in React resembles event handling in plain HTML/JS. Yet, there are differences: React events names use camel case, not a lowercase, and in JSX, you pass a function as the event handler, not a string.
The main problem is that different browsers handle events differently — what can be rendered in Google Chrome won’t necessarily be rendered in Internet Explorer. React solves this problem. It introduces synthetic events, which emulate the work of a native event in every browser.
7. What is the difference between controlled and uncontrolled components?
What answer to expect:
Controlled components are bound to values, and to introduce any changes, event-based callbacks should be used. The input in controlled components is handled by React, not by DOM, the mutable state is kept in the state property, and there are functions that control the data passed down to the component. Meanwhile, uncontrolled elements are very similar to the conventional HTML inputs, and the form data is handled by DOM. This data has its own state, which is updated with each change in input value.
8. How does context API work in React?
What answer to expect:
Usually, variables have to be passed from parent nodes to child nodes, which may be a cumbersome solution. Context is a way to share this data without passing it explicitly through each level of a tree. Context passes the data from a parent to every child of it, which is marked as a context consumer. In other words, a parent is a context provider, and each child that agrees to accept changes in context is a context consumer. For example, context is often used to set UI themes, which are used in most, if not all, parts of the app.
Programming Basics — React Interview Questions
Why to ask these React interview questions:
You should interview your candidate on programming basics to make sure that he doesn’t only build monotonous forms but is curious about programming in general, emerging technologies, and new tech challenges. After all, if you want them to develop their expertise, you need to make sure that they want it to, and the basic knowledge of programming proves it. Since there are many interview questions for a react developer to ask, it would be better to include the following ones as well:
9. What programming paradigms are you familiar with?
What answer to expect:
There are many programming paradigms, such as imperative, declarative, structured, procedural, event-driven, flow-driven, and others. But the two major paradigms are Object-Oriented Programming (OOP) and Functional Programming (FP).
10. What are the fundamental principles of Functional Programming?
What answer to expect:
There are seven essential principles of Functional Programming:
- Immutability — Once a value is assigned to something, this value shouldn’t change.
- Disciplined state — You should avoid shared, mutable states.
- Referential transparency — Once you replace a function call with its return value, the behavior of the app shouldn’t change.
- Pure functions — A function always has to return the same output if given the same inputs, and it should have no side-effects.
- First-class functions — Functions can be passed as arguments, returned as values, stored in data structures, and assigned to variables.
- Higher-order functions — Higher-order functions should take functions as arguments and returns a function as its output.
- Type systems — The compiler helps you avoid common development mistakes and errors.
11. What is idempotence and how it is connected with FP?
What answer to expect:
Idempotence is a concept used in mathematical logic and computer science. Simply saying, it means that the same operation should always return the same output if provided the same input. Its relation with the Functional Programming rests in the fact that the concept of idempotence reflects one of the core principles of FP, that is, Pure functions.
12. What is currying, side effect, and pure function?
What answer to expect:
Currying is an advanced technique of working with functions, which doesn’t call but transforms the function. It translates a function from callable as f(a, b, c) into callable as f(a)(b)(c).
Side-effect is any change in the application state, which modifies some state variable value outside its local environment.
Pure function is a function that (1) always returns the same output if given the same inputs and (2) has no side effects.
13. How do you understand the concepts of MVC, MVP, MVVM?
What answer to expect:
MVC is a software development pattern, which stands for Model, View, Controller.
MVP is a software development pattern, which stands for Model, View, Presenter.
MVVM MVC is a software development pattern, which stands for Model, View, View Model.
Models contain all the application data. Views display visual elements and controls on the user interface. Controller takes inputs and converts it into commands for Model or View. Presenter acts as a middleman between Model and View — it takes data from Model and formats it to be displayed by View. A View Model transforms data from Model into values that View can display.
JavaScript Basics — React Interview Questions
Why to ask these questions:
JS basics is another sphere you should interview your candidate on. Simple as it is, React can’t be mastered without the knowledge of the fundamental principles of JavaScript — the programming language using the React library. On the whole, common React interview questions include certain questions that come next:
14. What is the difference between primitives and non-primitives?
What answer to expect:
There are five primitive data types in JavaScript: number, string, boolean, undefined, and null, but there is one non-primitive data type: object. Primitive data types are stored by value, while non-primitive data types are stored by reference. When creating a primitive variable, you create a potential new address, but when you create a non-primitive object, you create a pointer to that object.
15. What is closure, and what is it used for?
What answer to expect:
Closure is a mechanism that connects a function and a reference to its outer scope. Created at the moment of function creation, a closure provides access to the outer scope from the function’s inner scope. A closure controls what is and what is not in the scope of a certain function. It can be used in event handlers, callbacks, currying, and other functional programming patterns. Most importantly, closures are often used for object data privacy.
16. What is the difference between a function and a plain object?
What answer to expect:
A plain object is a set of key/value pairs wrapped in {}, which is perfect for storing simple data sets. A function is a Function object; it has parameters, which are called arguments and passed to the function by value, and a return statement, which specifies the value that the function returns.
17. How does hoisting work in JavaScript, and what is the order of hoisting?
What answer to expect:
Hoisting is a JavaScript mechanism, which moves variables and function declarations to the top of their scope before code is executed. As for the order of precedence, variable assignments go first, function declarations go second, and variable declarations go third.
18. What is the difference in usage of callback, promise, and async/await?
What answer to expect:
Callbacks are the functions that take time to produce a result. These functions, however, prove ineffective when there are callbacks nested inside other callbacks. Promises are callbacks that have three states: resolved, rejected, or pending. They are more effective than callbacks. Yet, async/await is the most convenient way of dealing with asynchronous code. Once called, an async function returns a Promise. If it returns a value, the Promise is resolved with this value. If it throws an exception or a value, the Promise is rejected with the thrown value. An await expression located inside of an async function pauses the execution of it and waits for the Promise to be resolved, then, resumes the execution, and returns the resolved value.
19. How does the inheritance model in JavaScript differ from that in other languages?
What answer to expect:
JavaScript doesn’t support multiple inheritances. To inherit property values at run time, JavaScript searches through the prototype chain of an object to find a value. Each object has a single associated prototype, so JavaScript can inherit dynamically only from one prototype chain.
Front-End Development Basics — React Interview Questions
Why to ask these questions:
Today, developers have to be tech-savvy and be able to create interactive web applications. For companies to ensure that their candidates match those requirements, their potential workers have to be aware of the basics of the front-end development process.
In other words, front-end questions are a must for you to understand the development experience of a candidate. How deeply are they immersed in this sphere? Do they understand the general principles of modern front-end development?
20. What is an SPA? What are the major pros and cons of it?
What answer to expect:
SPA stands for a single-page application, and it is a web solution type that dynamically rewrites the current web page with new data from the client as contrasted to the default method when the browser loads entire new pages.
As for the advantages of SPAs, they are reactive, user-friendly, fast to load, easy to add advanced features, and more resource-efficient in terms of bandwidth usage. At the same time, they use a lot of browser resources and don’t perform quite well in terms of search engine optimization.
21. What is server-side rendering, and what problems does it solve?
What answer to expect:
Server-side rendering is a method that renders the React components on the server and brings HTML content as an output. It is the opposite of client-side rendering, where a browser downloads a minimal HTML page, renders the JavaScript code, and fills it out with the content. Server-side rendering solves the problem with search engine crawlers not understanding JavaScript, which is crucial for SEO results. Also, it can improve application performance, which is important for content-heavy websites.
22. What is CORS?
What answer to expect:
CORS stands for cross-origin resource sharing, and it uses additional HTTP headers, which make a browser grant a web app, which is running at one origin, access to some certain resources from a different origin. A cross-origin HTTP request is a request of resources from a domain, protocol, or port different from the origin domain, protocol, or port of the web app. For the sake of data security, browsers restrict cross-origin HTTP requests if they are initiated from scripts.
23. How do you create and manage user sessions properly? What is JWT?
What answer to expect:
User sessions stand for the series of user interactions with the app, which are tracked by the server. They are used to maintain user-specific states, that is, persistent objects and authenticated user identities. JWT stands for JSON Web Token. It is an open standard for access tokens creation, which is based on the JSON format. Usually, it is used to transfer data for authentication in client-server apps. To keep users logged in when they navigate through different screens, JWT is generated on a server and sent to a client, where it is transformed into a cookie. This cookie keeps the user logged in. To drop a session, you use a logout method when JWT is dropped from cookies.
Network Data Transfer Basics — React Interview Questions
Why to ask these React interview questions:
A front-end developer inevitably has to deal with the API. They have to know the basic principles of working with it to prevent technical errors and security risks.
24. What are the parts of an HTTP request?
What answer to expect:
An HTTP request message consists of the following parts: a request type, a series of HTTP headers or header fields, and a request body if needed.
25. What is the difference between HTTP2 and HTTP?
What answer to expect:
HTTP, which stands for Hypertext Transfer Protocol, is the foundation of data communication for the World Wide Web. HTTP has existed since the very dawn of the Internet, but five years ago, it was revolutionized, and HTTP2 was introduced. The goals of HTTP2 include a protocol negotiation mechanism, improved page load speed, compressed request headers, request multiplexing, request pipelining, Head-of-line blocking, etc..
26. What is REST and RESTful?
What answer to expect:
REST stands for a representational state transfer. It is a software architecture style, which introduces a set of constraints that should be used when creating web services. A web service that complies with REST standards is called RESTful.
27. What is a websocket, and how does it work?
What answer to expect:
Websocket is a computer communications protocol, which provides full-duplex communication channels over a single Transmission Control Protocol connection. This advanced technology enables bidirectional communication between a server and a browser — you can send messages to the server and get event-driven responses with no need to poll the server for a reply. A websocket starts as a normal HTTP request and response. Within this HTTP request-response chain, the client requests a websocket connection to be opened and awaits for the server to respond. If the server’s response is positive, a websocket connection between a server and a client is established. Data flows with the help if a basic framed message protocol, which is closed once both the server and the client decide to end the websocket connection.
28. What is a cookie, and what types of cookies are you familiar with?
What answer to expect:
A cookie is a small chunk of data, which is sent by a website and stored by the user’s browser. This data is used for a website to remember stateful information or to record users’ browsing activity. As for the types of cookies, there are session cookies, permanent cookies, same-site cookies, HTTP-only cookies, etc.
29. What is the difference between cookies and local storage?
What answer to expect:
Cookies and local storage are designed to serve different purposes: cookies are mostly read by the server-side, and local storage is read by the client-side. Also, local storage can store data of large sizes than cookies.
Security Basics — React Interview Questions
Why to ask these questions:
If you want your app to be secure, prevent attacks, leaks, and other data safety threats (of course, you do), you have to make sure that your developer knows what threats to expect and how to handle them. Therefore, it is vitally important to consider the questions that follow next:
30. What types of security attacks on the front-end are you aware of?
What answer to expect:
Major security concerns for a front-end developer are SQL injections, cross-site request forgeries, and cross-site scripting attacks.
31. What is brute force and how to avoid it? Have you heard about “salt and pepper” terms?
What answer to expect:
When somebody conducts a brute-force attack, they submit numerous passwords with the hope to eventually guess correctly. This attack can be used to break into a closed-access system or account or to decipher encrypted data. It is often used when the attacker sees no other weaknesses that they may tackle to breach the system. The simplest way to prevent brute-force attacks is to limit the number of failed login attempts. Using Captcha or two-factor authentication may also help.
As for salt and pepper, it is a password hashing terminology.
32. How can you protect your app from XSS?
What answer to expect:
To conduct a cross-site scripting attack, one injects a piece of malicious code, which runs a client-side script, into a legitimate web page. Once a user opens the infected website, the user’s browser downloads the script. To prevent an XSS attack, you should encode all variable strings before they will be displayed on the web page. In other words, you should convert every potentially dangerous character to an HTML entity. Also, you should limit input by types: a user can type only numbers into a number field and so on.
33. What do you know about CSRF?
What answer to expect:
CSRF stands for Cross-Site Request Forgery — this security attack forces a user to perform unwanted actions on a website. By doing a certain action, users can leak data, change the session state, or manipulate their own account without being aware of it. The malicious web request usually includes proper URL parameters, cookies, and other web data, so the server doesn’t recognize a forgery. By trusting an authorized user, the server executes the action they performed without asking to confirm it.
Code Testing Basics — React Interview Questions
Why to ask these questions:
Not to disrupt the development process and not to impose any extra headaches for the QA team, a developer has to test their code to identify and tackle errors before deploying the feature.
34. What is the difference between TDD and BDD?
What answer to expect:
TDD stands for test-driven development, and BDD stands for behavior-driven development. In both TDD and BDD, tests are written before development. Both TDD and BDD aim to prevent bugs, and both involve detailed documentation. Yet, the implementation of the two is different. In TDD, a developer writes an automated test case based on the project specifications. The development continues until this test is executed successfully. In BDD, a developer writes given-when-then expressions, which resemble tests but are written in simple English. Thus, TDD is aimed to test the feature implementation, and BDD tests feature behavior. Tests in TDD are written in a programming language, and tests in BDD are written in English.
35. What types of testing are you familiar with, and what is the purpose of each?
What answer to expect:
There are many different types of testing: manual and automated, functional and non-functional, scripted, exploratory, and adhoc. Among the functional types, there are unit, integration, system, smoke, regression, and acceptance testing. Among the non-functional testing types, there are UI/UX, compatibility, API, performance, security, and accessibility testing. A developer must know about these types: unit, functional, integration, end-to-end (e2e), and regression testing.
Check our article “How to Find Your Way Around Different Types of Software Testing?”.
36. How to manage e2e tests from the front-end perspective? What tools would you use for it?
What answer to expect:
To manage end-to-end tests from the front-end perspective, you can use such tools as Enzyme, Jest and jasmine, testcafe/puppeteer/nightwatch.
To Wrap Up
So, the React interview becomes easier to conduct when you know what React.js interview questions to ask and what answers to expect.
If you are a React developer, this article is your cheat sheet: having learned the answers to each of these React interview questions, you will most likely pass a technical interview at Keenethics.
If you are interested not in becoming a developer but in finding a perfect candidate for your team, we hope that this article becomes a cheat sheet as well. Just print it out and keep it within an easy reach when developing interview questions on React.js and conducting interviews. We hope that these ReactJS interview questions and answers will help you conduct a successful interview and easily understand if the candidate deserves to be a member of your team.
We will gladly let you choose from the talent pool we have here in Keenethics. Our specialists are experts in building websites using React. Learn more about React.js development services that we offer.